Writing a UR (User Registration) Module
This page outlines the steps that can be used to write a UR (user registration) module. UR modules are pluggable, standalone modules that are used to interface with the UR validation framework introduced in 1.1.6.4.
Basically, a UR module contains all the logic necessary for alternative methods of user registration validation, and utilizes method hooks in the framework to display registration fields and text, retrieve user input, approve/disapprove registration requests, and display appropriate confirmation and error messages. Designing a UR module should never require modification to the Wikka core code libraries and routines.
About this tutorial
This tutorial outlines the development of the URInviteCodeModule, a simple module that prompts for a predetermined code before a registration request is processed. The module ships with Wikka 1.1.6.4, and can be found in the <wikka-dir>/libs/UR directory. If you are following thee tutorial, you might want to move/rename the URInviteCodeModule directory so you have the original to compare with if you run into problems.
Conventions
All file references will be with respect to your top-level installation directory (the directory which contains the folders/subdirectories 3rdparty, actions, handlers, etc.). The term "folders" and "subdirectories" are used interchangeably. As an example, the UR folder that is located in the top-level libs folder will be shown as libs/UR/.
I develop on a Mac iBook using the UNIX command line, so you might have to modify your commands accordingly for other platforms.
Setting up a new module (URInviteCodeModule)
Modules must reside in the libs/UR/ folder. Start by creating a new folder:
mkdir libs/UR/URInviteCodeModule
Make libs/UR/URInivteCodeModule your current directory. From this point on, the tutorial will make the assumption that you've done this step.
cd libs/UR/URInviteCodeModule
We'll use the URDummyModule as a template, so let's copy that into our new module folder from the libs/UR/URDummyModule folder:
cp ../URDummyModule/URDummyModule.php URInviteCodeModule
Extending the URAuthTmpl class
All modules must extend the URAuthTmpl class. So the first step is to change the class declaration to reflect the name of your new class. Change this line:
class URDummyModule extends URAuthTmpl
to this:
class URInviteCodeModule extends URAuthTmpl
Creating the constructor
The constructor function will also need to be changed to reflect the new name you've given your class...so modify the constructor:
function URDummyModule(&$wakka) { ... }
to:
function URInviteCodeModule(&$wakka) { ... }
The main purpose of the module constructor is to initialize the parent with the current $wakka instance and to initialize any parameters that are needed for your specific module. Any parameter added to wikka.config.php will be available to your module via the $wakka->config array. The UR framework will handle details involving instantiation of your module and multiple modules defined in the UR_validation_modules parameter, so you should never have to access this in your code.
Our module requires one parameter: The invite code that the user will have to match in order for site registration to be successful. We create a new instance variable to hold this value:
class URInviteCodeModule extends URAuthTmpl { var $inviteCode;
and initialize this variable inside the URInviteCodeModule constructor:
function URInviteCodeModule(&$wakka) { $this->URAuthTmpl($wakka); if(isset($wakka->config['UR_InviteCode_password'])) { $this->inviteCode = $wakka->config['UR_InviteCode_password']; } }
URAuthTmplDisplay()
When UserSettings is first called by the browser, the underlying action (actions/usersettings.php) makes a call to a hook function called URAuthDisplay(). In turn, the framework passes this call on to any registered modules. Therefore, if you want your module to output form elements, you will need to define an instance method called URAuthTmplDisplay(). (Currently, this call is made between the creation of the Login-related form fields [Your WikiName, Password] and the Register-related form fields [Confirm password, Your e-mail address]. Plans are to include additional function hooks to allow for placement of output elsewhere on the generated page.)
We have need of only one input field: A field for the user to enter the invite code. Any error messages should be displayed at this time as well.
function URAuthTmplDisplay() { if(!isset($this->inviteCode)) { echo '<em class="error">Error in URInviteCode module</em>'; return; } echo "Enter invitation code: <input type='textarea' name='UR_inviteCode'/><br/>"; }
The "error" class is a Wikka-specific class used for styling errors throughout the application; I recommend using this same styling for your own error messages. The name of the input field (UR_InviteCode) will be available to your module via the $_POST array.
URAuthTmplVerify()
When a user clicks the Register button, several validation checks are made against the user-entered data by usersettings.php. Prior to any of these checks being made, the action class calls URAuthVerify(), which in turn calls URAuthTmplVerify() on every registered UR module. Return values from each module are ANDed together, so all registered UR modules must return TRUE from URAuthTmplVerify() before validation continues.
URAuthTmplVerify() must return only one of two values: boolean FALSE (registration is invalid) or boolean TRUE (registration is valid). Remember that the usersettings action will continue to carry out its internal input validations and might override your module's TRUE return value.
We consider a registration to be valid if the user enters the correct invite code. Therefore, we will compare the user's input with the correct invite code, and return TRUE if the values match, FALSE otherwise:
function URAuthTmplVerify() { if(!isset($this->inviteCode)) { return false; } if(isset($_POST['UR_inviteCode']) && $_POST['UR_inviteCode'] === $this->inviteCode) { return true; } return false; }
Note that as a failsafe, we simply return FALSE if no conditions are evaluated, which is probably favorable over returning TRUE when we haven't correctly anticipated all possible cases.
Also, remember that after the user POSTs with the Register button, your module is reinstantiated, and previous instance variable values are lost. This is important if you plan on comparing data that is derived or created in URAuthTmplDisplay() with user-entered values in URAuthTmplVerify(). You will probably have to persist the data in a client-side or server-side (session) cookie so that the data is accessible in subsequent POSTs. This topic is beyond the scope of this tutorial. The URCaptchaModule offers a working example of how this might be accomplished.
Implementation and testing
To test your new module, simply create the following parameters in your wikka.config.php file:
'UR_validation_modules' => 'URInviteCodeModule' 'UR_InviteCode_password' => 'some_invitation_code'
Reload your UserSettings page (you will need to logout first). You should see output similar to the following:
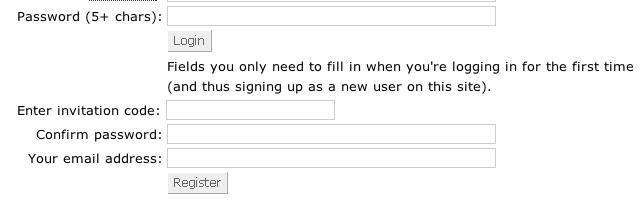
The framework will generate a generic error message if registration is disapproved by any registered module, so for this module, we simply chose to use this generic error for user notification:
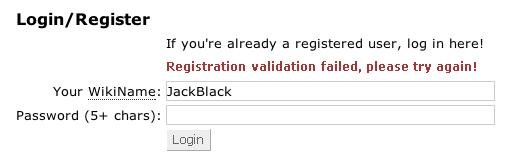
Successful entry of the invite code should generate a successful registration:
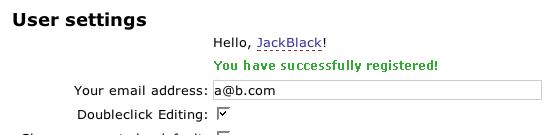
CategoryURModules